General Instructions
Create and Use a new Branch hw12
We will create a new git branch called hw12
for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 11. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw12
. Review the section on submission for using push with a new branch.
Use README.md
for Answers
You will modify the README.md
file to contain the answers to this homework.
# Homework #12 Solution
**Your name** **NetID: yourNetID**
Questions
Tips: We are integrating the client App and the server. You will need to login frequently. Set your browser to remember your club login for development.
Question 1. (15 pts) Members and Activities DataBases
We will use promises with NeDB so use the nedb-promises in your implementation, i.e., you need to install it in your clubServer directory.
(a) Create Initial Activity and Members Databases
Write a small Node.js program called initDataBases.mjs
to initialize the activities and members NeDB databases from your events and (hashed) users JSON files. Be sure to remove existing database entries prior to inserting so you can get the database to a consistent state by re-running this program as often as you need. Let NeDB assign unique Ids for your activities and members. Show your code here.
(b) Integrate Member Database Into Server
Use the member data base created in part (a) for all parts of your code that involves members/users. Remove previous code that read in member information from a JSON file. You show your updated “login” and get “member” handling functions here.
(c) Integrate Activity Database into server
Use the activities data base created in part (a) for all parts of your code that involves activities. Remove previous code that read in activity information from a JSON file. You show your updated interfaces for getting, adding, and deleting activities. For the delete interface have it take and use the _id
assigned to determine the item to delete (rather than an array “index” which no longer applies). Show your updated code here.
Question 2. (10 pts) Testing Server with DataBases
(a) Login Tests
Do you need to modify your Mocha based login tests from homework #11? Perform any modifications and show a screenshot of running your login test against the updated server from problem 1.
(b) Activity Tests
Do you need to modify any of your Mocha based activity tests from homework #11? Show code changes here and a screenshot of your activity test results.
Question 3. (10 pts) Proxies in Development
(a) GUI and Server development
You need to understand that there is a development server used by Parcel to serve your React app and a separate server for your club that you have been developing. Answer the following questions:
- Start up your clubReact development bunder. What host and TCP port is the Parcel bundler running on? Write the information here.
- In a separate terminal start up your clubServer. What host and TCP port is your server running on? Write the information here. (Note that this cannot be the same as above)
(b) Proxy Configuration
The Parcel bundler has the option to proxy requests as explained in API proxy. If we try to make a fetch call from our React app while in development it will go to the Parcel development server rather than your clubServer. To have Parcel forward these requests you must create a .proxyrc.json
file and put it in your clubReact directory. For example to forward a request to the /members
path on I would use a file like:
{"/members": {
"target": "http://localhost:7761"
} }
Update this file and show it here to pass on requests to get activities based on your server implementation (path and port).
Question 4. (15 pts) Fetch for Activities
Now lets get the activities data for members from our server rather than a development JSON file!
(a) Members Activities Component
You should have created a simple activities component to show your activities to members and/or guests. However we want to use the life cycle method componentDidMount
as the place to make the fetch call. If you Activities component is not a class based component update it here with its state containing the an empty array of activities.
(b) Fetch Activities in the Component
Add the component life cycle method componentDidMount
and use fetch to get the list of activities from the server. Take a screenshot that shows the network panel with the fetch to activities and some of the data returned. My screenshot looks like:
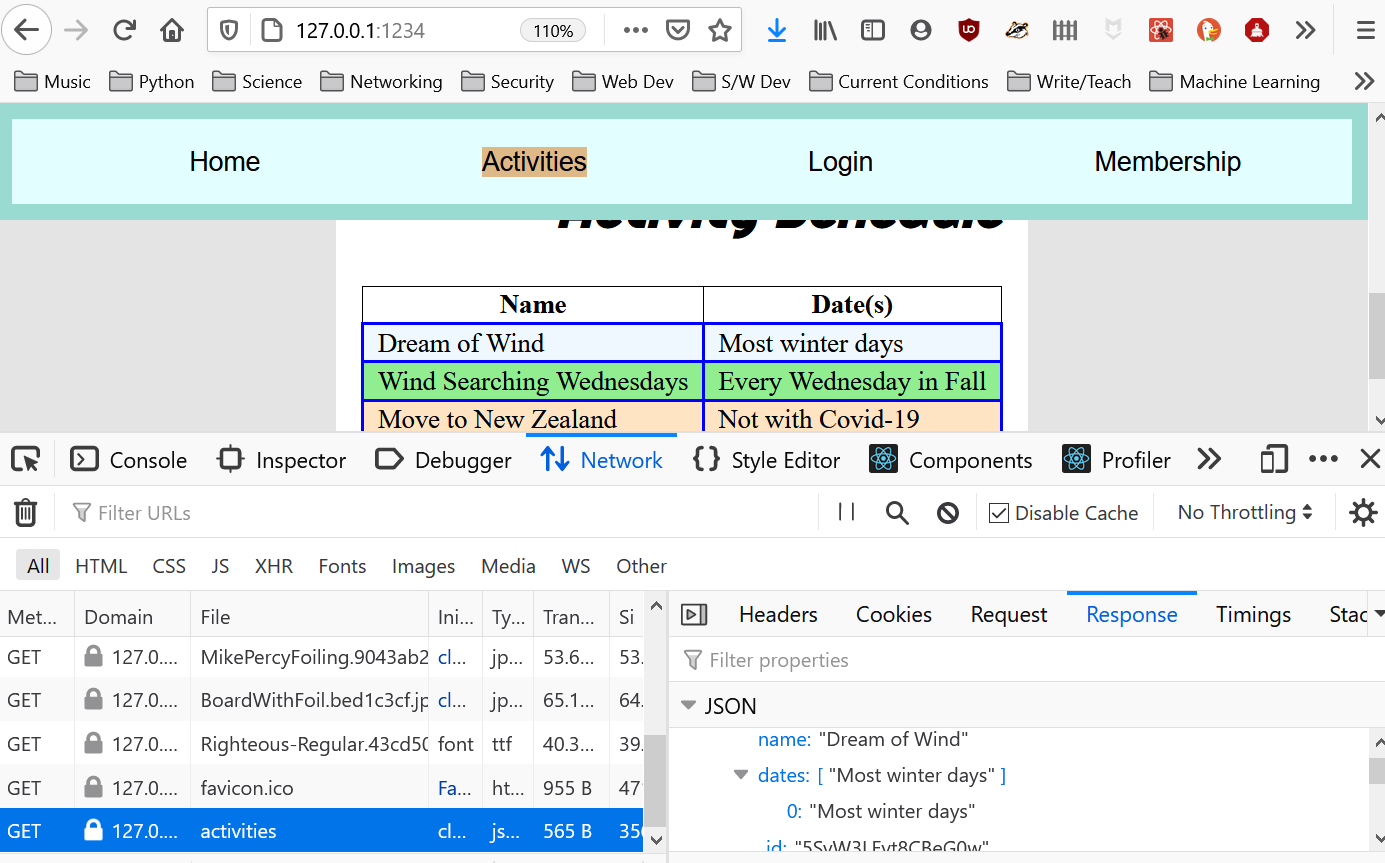
Show your updated Activities component code here.