General Instructions
Create and Use a new Branch hw11
We will create a new git branch called hw11
for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 10. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw11
. Review the section on submission for using push with a new branch.
Use README.md
for Answers
You will modify the README.md
file to contain the answers to this homework.
# Homework #11 Solution
**Your name** **NetID: yourNetID**
Questions
For JSON-Schema validation we will use the ajv library. See the course notes for references on JSON Schema and visit the ajv page at NPM for usage instructions.
Question 1. (15 pts) Testing
We are going to use the Mocha test framework along with Chai as described in the course Testing slides. Within your clubServer
directory create a test
subdirectory to hold all your test files.
(b) Testing Activities Interfaces
We are going to change our club’s policies a bit to give us a bit more interesting test scenarios. We will now allow members to add activities, but only admins to be able to both add and delete activities. Update your server code using the appropriate middleware on both the add and delete interfaces. Create an new testActivity.js
file in your test
directory and write tests for the following
- Get Activity Tests
- An array of activities is returns
- Add Activity Tests
- Try adding activity without logging in (should fail)
- Login as a member and add activity (should pass)
- Delete Activity Tests
- Try deleting activity without logging in (should fail)
- Login as member and Delete an activity (should fail)
- Login as admin and Delete an activity (should fail)
Show the output from the Mocha test framework.
Question 2. (10 pts) JSON Schema
(a) Activity Data Schema/Validation
Create a JSON schema to check the validity of “activity” data that would be sent to the server. Show an example of activity data and show the schema here. Add validation checking to the server where appropriate. Note you will be testing validation checking in question 4.
Question 3. (15 pts) Applicant Interface, JSON Protection, Schema
(a) JSON Protection
We will need to protect our server from bad inputs (intentional, injection attacks, and unintentional). A very first step is to limit the size of the JSON for the activity we will accept. Look at the options for the express.json middleware for how to set this. Next we need some error handling middleware. This need to take the form:
function jsonErrors(err, req, res, next) {
// prepare and send error response here, i.e.,
// set an error code and send JSON message
console.log(JSON.stringify(err));
return;
}
This handler (that you need to complete) goes after all middleware for dealing with the different POSTs including your handler code. Show your updated code here after you finish testing it in question 3.
(b) Applicant Data Schema
Your club had an application form that took specific information from applicants. Show an example valid JSON object representing this data. Create a JSON schema for this data and show it here.
(c) Application Interface
Add a new applicants API to your server at path /applicants
, that uses a POST method, and that takes data in the format you came up with in the previous problem. Use the AJV library to input check submitted JSON. Note that this is an “unprotected” interface. Show the code you added for this interface here. You do not need to do anything with the applicants data besides checking it.
Question 4. (10 pts) Testing Validation/Protection
Now lets test our new protection and validation code. We’ll test the new applicant interface first since it doesn’t require any login.
(a) Applicant
Write a new Mocha based test file to test for at least the following:
- Valid data is accepted
- Overly Large JSON data is rejected
- Reject data with missing required fields
- Reject data with bad email
Show a screenshot of your test results mine looks like:
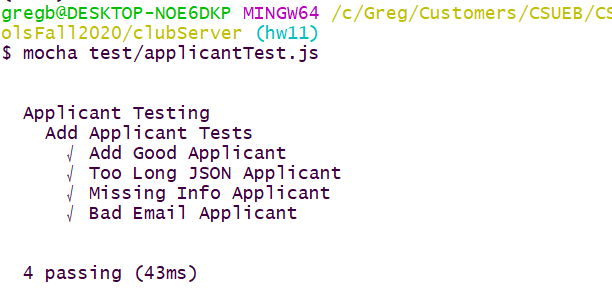
(b) Activity Add
To your existing activity tests add tests for:
- Overly Large JSON data is rejected
- Reject data with missing required fields
Show a screenshot of your test results and server debug messages. Mine looks like:
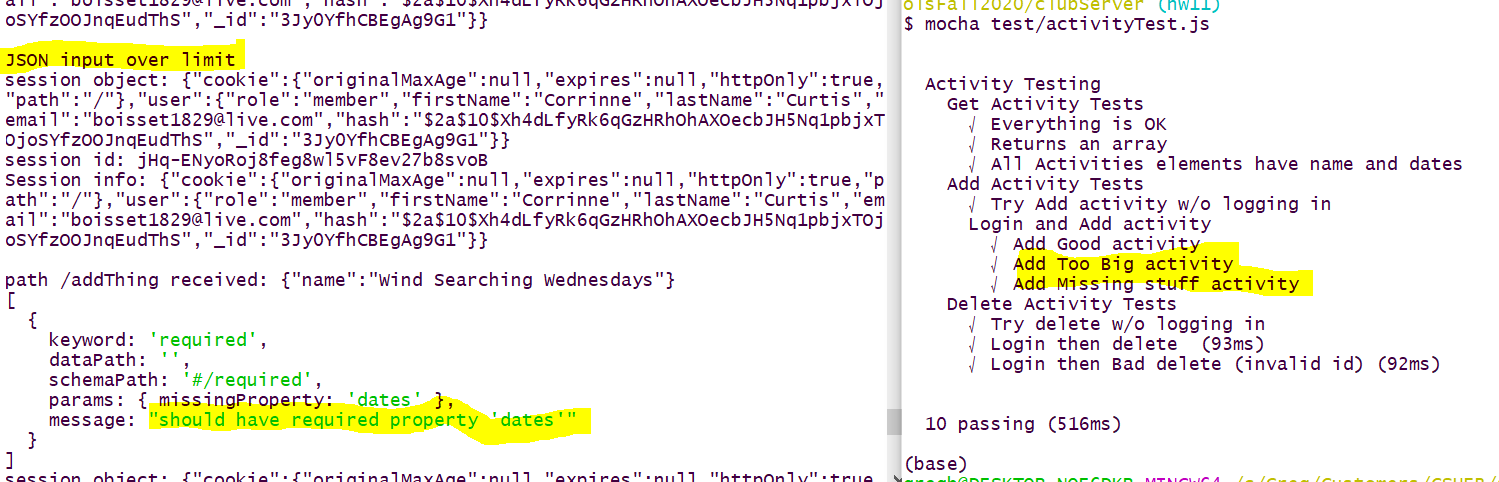