General Instructions
Topics this week: More React Class Components and interactivity. Networking.
Create and Use a new Branch hw7
We will create a new git branch called hw7
for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 6. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw7
. Review the section on submission for using push with a new branch.
Use README.md
for Answers
You will modify the README.md
file to contain the answers to this homework.
# Homework #7 Solution
**Your name** **NetID: yourNetID**
Questions
To receive full credit for problems 1-3 your deployed code/app must work.
Question 2. (10 pts) Membership Application Component
We are going to upgrade our “club application” function based React component to be a React class component so we can easily gather up the various “form” fields from the user. We still don’t have a server to send to so we will demonstrate functionality with a message “modal dialog” when the user click the “apply” button. However, we will do all our processing including adding/removing CSS classes via React. This will get up practice prior to the more complicated user interface for managing activities.
(a) Convert to class and Set up state
Convert your membership application component, i.e., the one with the “form” to apply to the club, from a React function component to a React class component. Add properties in the state for each input, selection, text area widget in your form. Show the JavaScript code for the constructor only here.
(b) Add handlers for input/change events
For each “form widget” create a handler function to deal with input or change events as appropriate and use these to update the components state. Make sure these work by looking at the “Components” React developer plugin. Show the JavaScript for these event handlers here and take a screenshot of the developer tool panel showing the components state. My screenshot looks like:
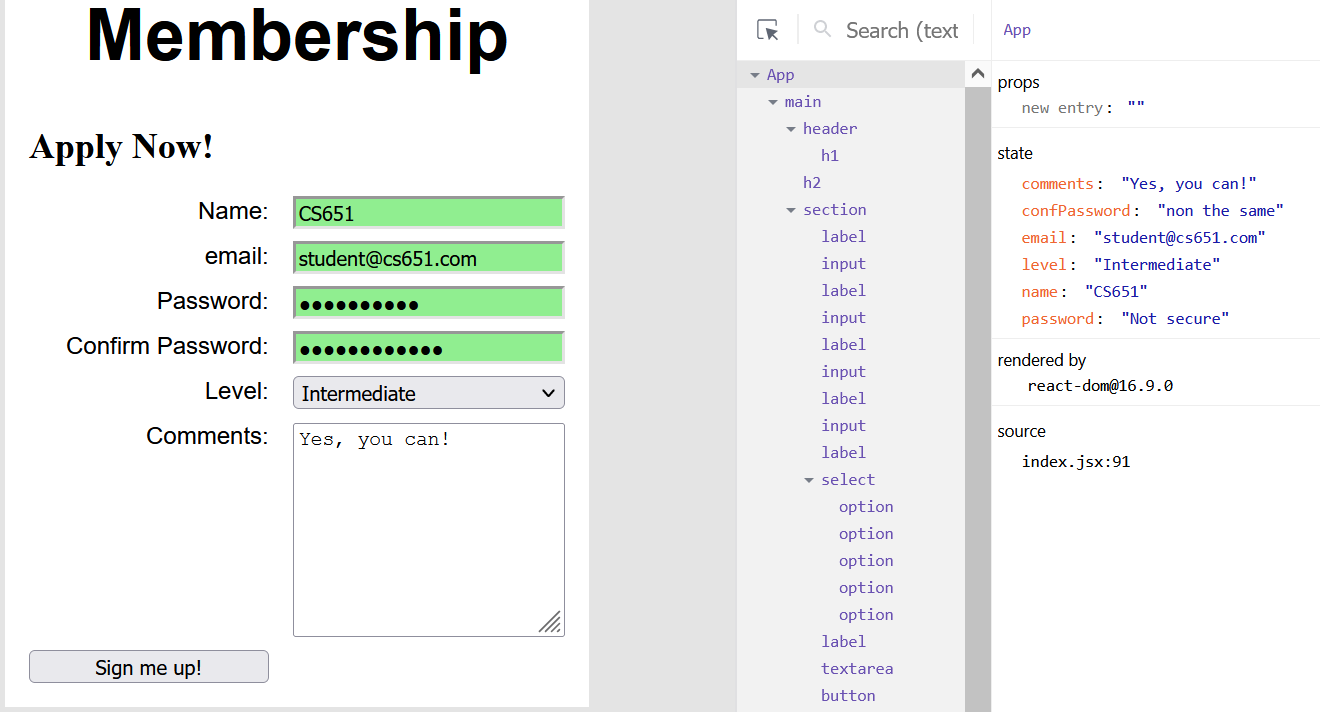
(c) Thanks Section
Below the form inputs add a <section>
to either thank the user for signing up or give an error message if there is a problem with a form field. Take screenshots on error and success. I get:
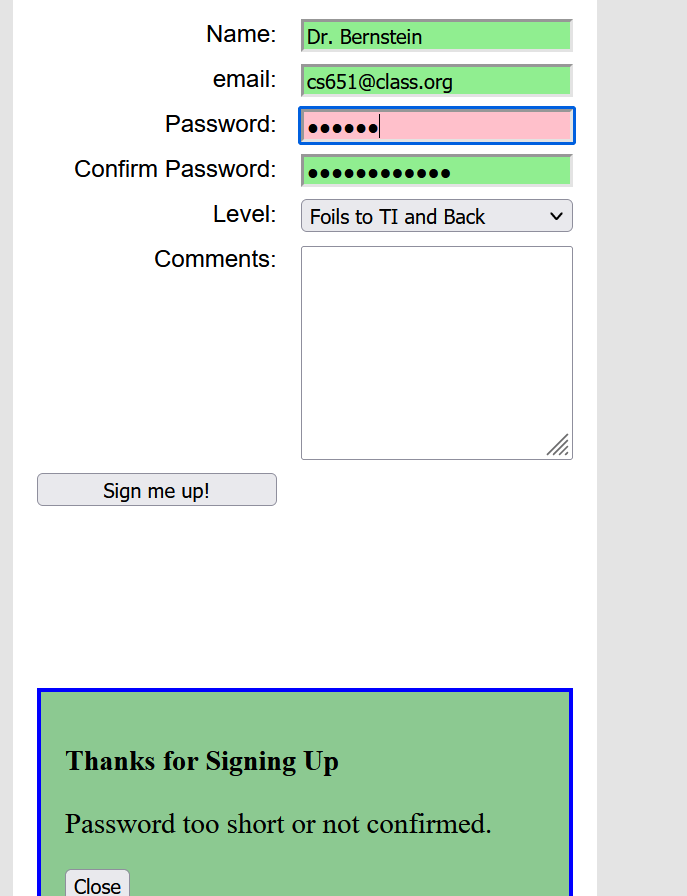
and
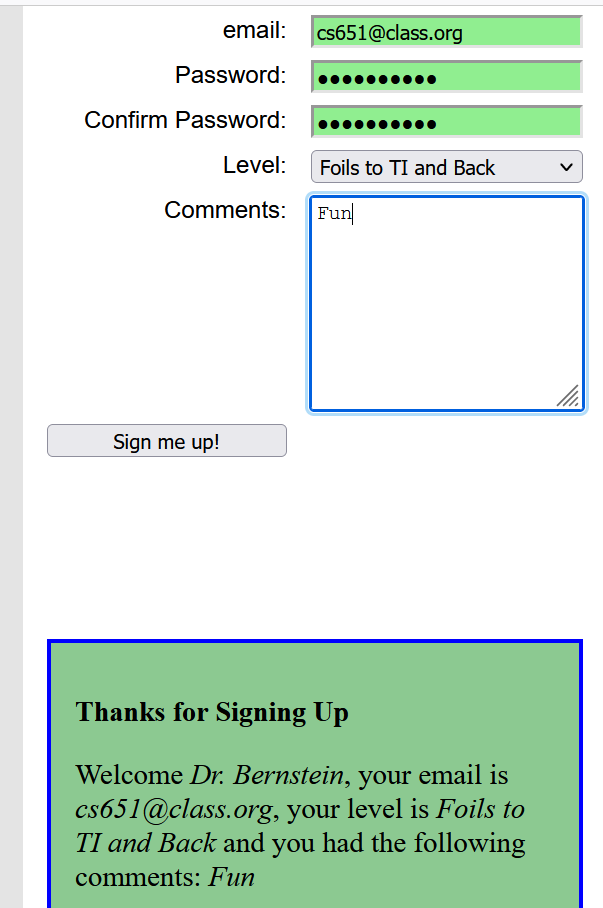
(d) Thanks Modal Dialog
Now by adding some more state (see course slides on interactivity) and the appropriate CSS classes make the <section>
in part (c) display like a “modal dialog” box when the “sign me up” button is click. Show the JSX code and CSS classes just for this section and take a screenshot. I get something like:
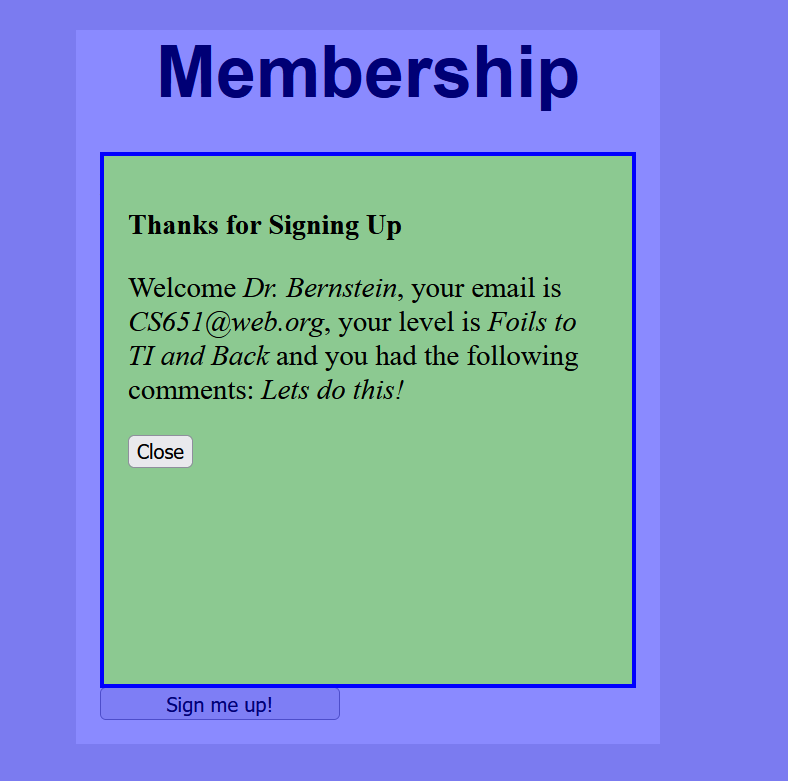
Question 3. (15 pts) Activity Management
We are going to create a component to allow administrators or members to add and delete club activities.
(a) Club Activity Management Mockup
Create a new component for club activity management. Call it AdminActivity.js
. “Mock up” the user interface to include functionality for adding and deleting club activities, but don’t provide any functionality yet. Take a screen shot. My screen shot looks like the following:
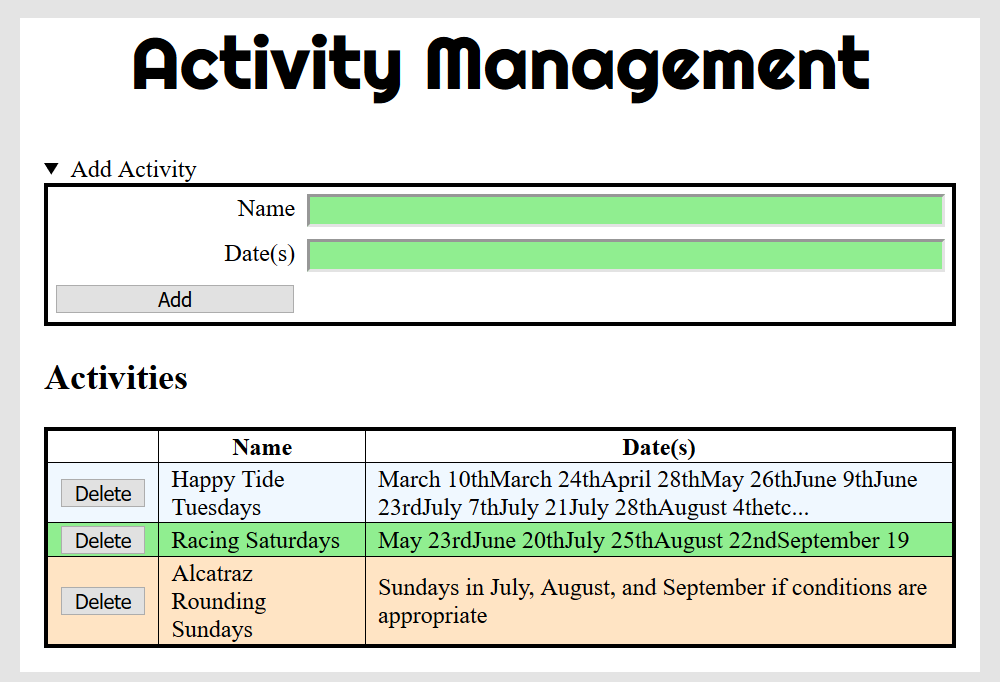
(c) Add Activity Functionality
For the UI mockup it didn’t matter if the React component was function or class based. Now we want to keep the activity information as state and manipulate it. What kind of component should we use? Add the capability to this component to add an club activity. Take a screen shot after you have added a couple activities and show the react component view. I get something like:
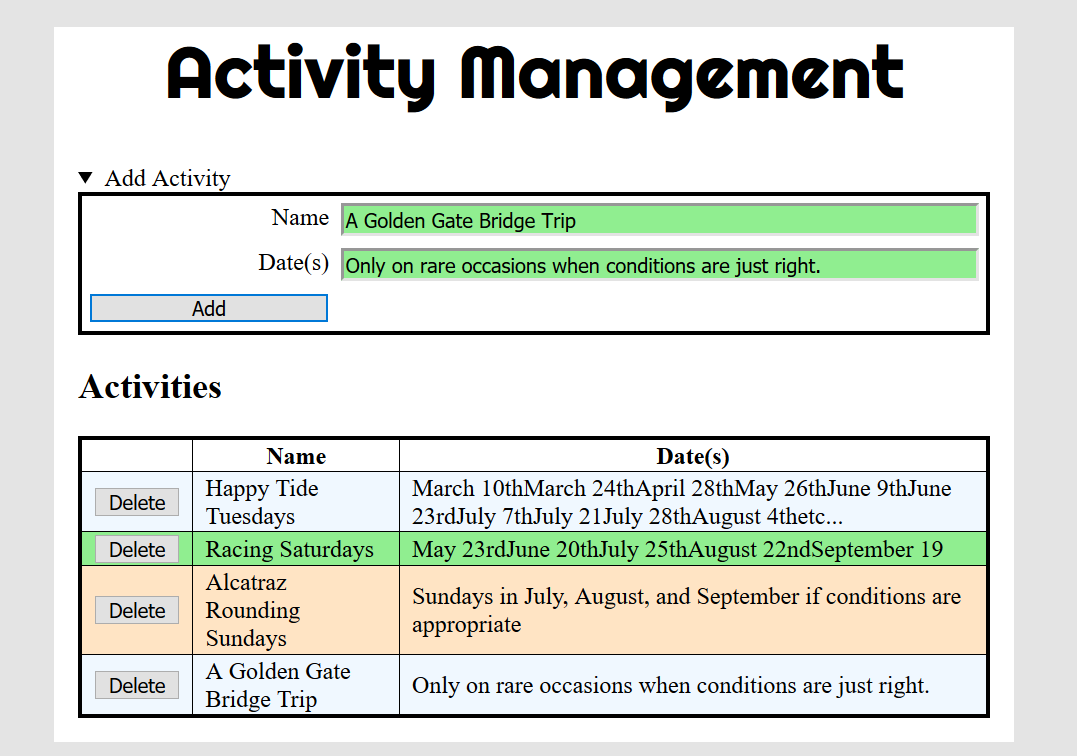
Notes We will not have this component get its activities from the App
component since in our final version this component will talk directly to the server. Hence you can have this component import some JSON data directly from a JSON file for development purposes. You do not need to let the App
component know about changes to “activities”.
(d) AdminActivity Component Constructor.
Show the code for your component constructor function here.
(f) Show Event handling functions
Show the event handling functions you needed to write.
Question 4. Deleting Activities (15 pts)
(a) Functionality to Delete Activities
Now give the admin user the ability to delete club activities. Demonstrate it here using before and after screen shots. I get something like:
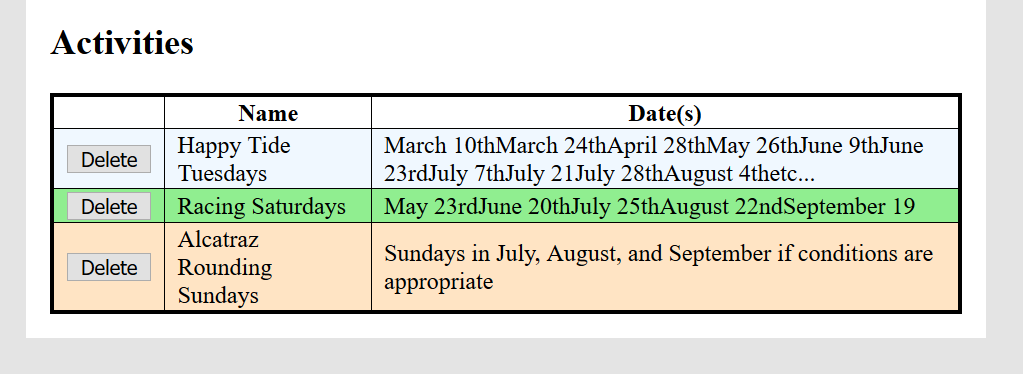
(b) Show Modification to JSX
Show modifications to your JSX to support activity deletion. Show subcomponents if you used them.
(c) Show Event Handler
Show your event handler to for the “delete” buttons here.
(d) Deploy to Server
Do a production build and deploy to a server. Put the link here.